- Designing algorithms is a skill that must be developed and when designing algorithms, mistakes will be made
- There are two main types of errors that when designing algorithms a programmer must be able to identify & fix, they are:
- Syntax errors
- Logic errors
Identify Errors in Algorithms (OCR GCSE Computer Science)
Revision Note
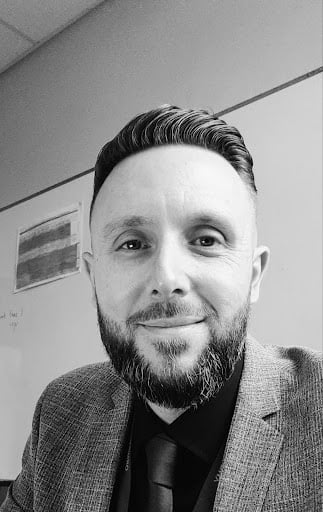
Author
Robert HamptonExpertise
Computer Science Content Creator
Syntax Errors
What is a syntax error?
- A syntax error is an error that breaks the grammatical rules of a programming language and stops it from running
- Examples of syntax errors are:
- Typos and spelling errors
- Missing or extra brackets or quotes
- Misplaced or missing semicolons
- Invalid variable or function names
- Incorrect use of operators
- Incorrectly nested loops & blocks of code
Examples
Syntax Errors | Corrected |
|
|
|
|
Logic Errors
What is a logic error?
- A logic error is where incorrect code is used that causes the program to run, but produces an incorrect output or result
- Logic errors can be difficult to identify by the person who wrote the program, so one method of finding them is to use 'Trace Tables'
- Examples of logic errors are:
- Incorrect use of operators (< and >)
- Logical operator confusion (AND for OR)
- Looping one extra time
- Indexing arrays incorrectly (arrays indexing starts from 0)
- Using variables before they are assigned
- Infinite loops
Example
- An algorithm is written to take as input the number of miles travelled. The algorithm works out how much this will cost, with each mile costing £0.30 in petrol. If this is greater than £10.00 then it is reduced by 10%.
Logic errors | Corrected |
|
|
Commentary | |
|
Worked example
Nine players take part in a competition, their scores are stored in an array. The array is named scores and the index represents the player
Array scores
Index | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 |
Score | 7 | 9 | 2 | 11 | 8 | 4 | 13 | 10 | 5 |
The following program counts the total score of all the players
for x = 1 to 8
total = 0
total = total + scores[x]
next x
print(total)
When tested, the program is found to contain two logic errors.
Describe how the program can be refined to remove these logic errors [2]
How to answer this question
- A common logic error if an algorithm contains a loop is checking it loops the correct amount of times, how many times should this algorithm loop?
Answer
- For loop changed to include 0
- total = 0 moved to before loop starts
Guidance
- Moving total outside the loop is not enough, it could be moved after the loop which would still be a logic error)
- Corrected code accepted
total = 0
for x = 0 to 8
total = total + scores[x]
next x
print(total)
You've read 0 of your 0 free revision notes
Get unlimited access
to absolutely everything:
- Downloadable PDFs
- Unlimited Revision Notes
- Topic Questions
- Past Papers
- Model Answers
- Videos (Maths and Science)
Did this page help you?